Today I’m going to make a small program that checks if a number is a palindrome in Python and in Java. There’s not really a clear reason that I’m doing this, it’s just an exercise to see the difference between Python and Java.
A palindrome is when a number or string is the same whether you read it from left to right or from right to left. Examples of a few palindromes are: 151, 22522 and 989. And 123 is not a palindrome because it’s different backwards.
Palindrome in Python
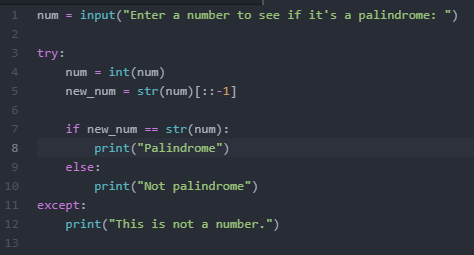
A short explanation of the code: we ask the user to enter a number. Then we try to change the input to an integer to see if the user really entered a number. If the input is not a number it can’t be changed to an integer and the code in the ‘except’ section is executed. If the user did enter a regular number it is converted to a string again and reversed using [::-1]. In Python [::-1] can be used to reverse strings.
Finally the reversed number is compared to the original number and if it is the same, it’s a palindrome.
Palindrome in Java
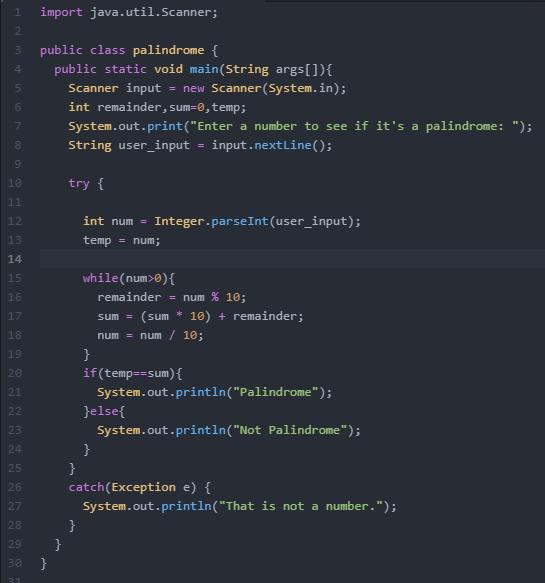
The formula for reversing the number in the code above is from javatpoint.com:
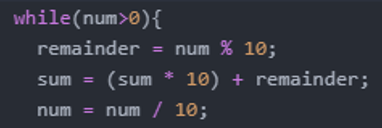
It’s slightly different from the one in the Python code and reverses the number digit by digit. It’s not important to understand but this is what I understand of it if you are interested:
The number is divided by 10 and the remainder is put at the beginning. Then the proces repeats so that each number that is at the end is moved to the front. So when you divide 151 by 10 the remainder is 1. Sum is now 1 and num is divided by 10, so num is now 15.1. Then a new loop starts: 15.1 % 10 is 5.1 so the remainder is 5.1. Sum is now 1 x 10 + 5.1 = 15.1. Num is again divided by 10 and is now 1,51. This is still above 0 so it loops again. But 1,51 % 10 is 0. So now sum is 15.1 x 10 + 0 = 151. This is exactly the same as the original number.
The concept for the rest of the program is the same: comparing the reversed number to the original number and if it’s the same, it’s a palindrome.
Instead of ‘try / except’ in Python, now it’s ‘try / catch’. Instead of print() it’s System.out.print(). The code for getting user input is also different. In Python you can just use input() but in Java you first need to import a scanner and then initiate it with Scanner [name] = new Scanner(System.in).
Lastly, in Python you can change a string to an integer using int(). In Java you can do this using Integer.parseInt().
Conclusion
In my opinion the Java code for the palindrome was more complicated than the Python code, but this may be a matter of the experience you have with both languages. In Python there are more functions for getting user input, changing a string to an integer etc. that are ready to use. Because of this the code tends to get a bit longer in Java and may look more complicated.
Sources
- https://www.javatpoint.com/palindrome-program-in-java
- https://www.w3schools.com/java/java_try_catch.asp
- https://www.geeksforgeeks.org/ways-to-read-input-from-console-in-java/
- https://www.javatpoint.com/java-string-to-int